Model Context Protocol (MCP) is actually mcp is actually a concept that is a few months old, but getting so hot nowadays as AI developennt evolves day by day. Let me look at what is Model Context Protocol (MCP) and deep dive into Model Context Protocol (MCP) with tutorial with implementation code examples.
What is Model Context Protocol (MCP)
Model Context Protocol (MCP), an open source project run by Anthropic, is an emerging framework that enables structured and dynamic context management for machine learning models, particularly in applications involving conversational AI, large language models (LLMs), and multi-modal systems.
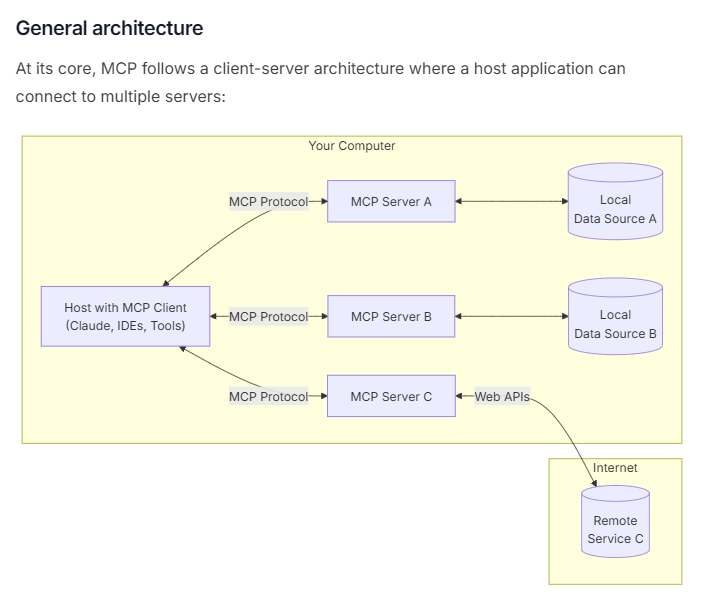
This protocol is designed to provide better control, efficiency, and adaptability in real-time AI applications by managing the context dynamically based on user interactions and data flows.
Key Features of Model Context Protocol
- Dynamic Context Switching – Enables AI models to switch between different contexts based on predefined rules or user interactions.
- Memory Management – Efficiently handles long-term and short-term memory for contextual conversations.
- Optimized for LLMs – Reduces token usage by maintaining only relevant context.
- Multi-Agent Coordination – Enables collaboration between multiple AI agents.
- Fine-Tuned Context Retrieval – Ensures only necessary context is fetched, improving response relevance.
Use Cases of Model Context Protocol
- Conversational AI – Enhances chatbots and voice assistants by dynamically managing conversation memory.
- Code Generation and Assistance – Allows AI coding assistants to remember user-specific coding preferences.
- Customer Support AI – Manages ongoing support cases by maintaining relevant conversation history.
- Medical AI Assistants – Helps AI-powered diagnostic tools maintain patient history context.
- Multimodal AI Applications – Enables AI models to understand and switch between text, images, and audio contexts.
Model Context Protocol in Action
Let’s explore MCP through practical examples with Python-based implementations.
1. Implementing a Basic Model Context Protocol
Here’s how you can implement a simple context-aware AI model using Python:
class ModelContextProtocol:
def __init__(self):
self.context = {}
def update_context(self, user, message):
if user not in self.context:
self.context[user] = []
self.context[user].append(message)
if len(self.context[user]) > 5: # Limit memory to the last 5 messages
self.context[user].pop(0)
def get_context(self, user):
return self.context.get(user, [])
# Example Usage
mcp = ModelContextProtocol()
mcp.update_context("User1", "Hello, AI!")
mcp.update_context("User1", "Can you help me with Python?")
print(mcp.get_context("User1")) # Output: Last 5 interactions
2. Implementing Context-Aware LLM using OpenAI GPT
MCP can be used to enhance OpenAI’s GPT models by feeding only the relevant conversation history.
import openai
class ContextAwareGPT:
def __init__(self, api_key):
self.api_key = api_key
self.context = {}
def update_context(self, user, message):
if user not in self.context:
self.context[user] = []
self.context[user].append(message)
if len(self.context[user]) > 5: # Limit history
self.context[user].pop(0)
def generate_response(self, user, new_message):
self.update_context(user, new_message)
context_str = " ".join(self.context[user])
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[{"role": "system", "content": "You are an AI assistant."}] +
[{"role": "user", "content": msg} for msg in self.context[user]]
)
return response["choices"][0]["message"]["content"]
# Example Usage
gpt_context = ContextAwareGPT("your-api-key")
response = gpt_context.generate_response("User1", "How do I install NumPy?")
print(response)
3. Implementing Multi-Agent Model Context Protocol
In complex applications, multiple AI agents can collaborate using shared contexts.
class MultiAgentMCP:
def __init__(self):
self.contexts = {}
def update_agent_context(self, agent, message):
if agent not in self.contexts:
self.contexts[agent] = []
self.contexts[agent].append(message)
if len(self.contexts[agent]) > 10: # Limit memory
self.contexts[agent].pop(0)
def get_agent_context(self, agent):
return self.contexts.get(agent, [])
# Example Usage
mcp_agents = MultiAgentMCP()
mcp_agents.update_agent_context("Agent1", "Analyzing data patterns.")
mcp_agents.update_agent_context("Agent2", "Generating a summary.")
print(mcp_agents.get_agent_context("Agent1")) # Output: Context history for Agent1
Advanced MCP: Using Vector Databases for Context Storage
Instead of storing plain text, a vector database like Pinecone or FAISS can be used to store and retrieve context efficiently.
Example: Using FAISS for Context Retrieval
import faiss
import numpy as np
class VectorizedContext:
def __init__(self, dim=128):
self.index = faiss.IndexFlatL2(dim)
self.context_data = {}
def add_context(self, user, vector):
self.index.add(np.array([vector]).astype('float32'))
self.context_data[len(self.context_data)] = (user, vector)
def retrieve_context(self, vector, k=3):
_, indices = self.index.search(np.array([vector]).astype('float32'), k)
return [self.context_data[idx] for idx in indices[0] if idx in self.context_data]
# Example Usage
context_manager = VectorizedContext(dim=128)
context_manager.add_context("User1", np.random.rand(128)) # Adding a random vector as context
print(context_manager.retrieve_context(np.random.rand(128))) # Retrieve similar contexts
Future of Model Context Protocol
- Integration with Blockchain – Ensuring secure context storage.
- Real-Time Context Compression – Optimizing large-scale AI models.
- Personalized AI Models – Tailoring responses based on user behavior.
Model Context Protocol (MCP) : Final Words
Model Context Protocol is transforming AI by improving how models understand, store, and retrieve context dynamically. Whether for chatbots, LLMs, multi-agent AI, or vectorized knowledge bases, MCP enhances efficiency and responsiveness.